1. PROJECT: One Life
1.1. Introduction
This project portfolio details my key contributions to the project One Life and serves to document the key features I implemented.
One Life is a desktop application designed by me and other students from the National University of Singapore (NUS) as part of a requirement for our software engineering module under the given constraints that the user must interact with it using a Command Line Interface(CLI).
One life is used for storing, updating, and displaying data related to patients and doctors. The user interacts with it using a CLI, and it has a Graphical User Interface (GUI) created with JavaFX. It is written in Java, and has about 10 kLoC.
1.2. Summary of Contributions
-
Major enhancement: added the ability to update and display medical records.
-
What it does:
-
Enables the user to update the current medical record history of a specified patient on a specified date.
-
Checks if the given information such as the date, diagnosis or treatment are valid.
-
-
Justification:
-
Streamlines a key part of medical operations in a hospital. (Updating and referring to the current medical history of any given patient.)
-
-
Highlights:
-
Improves how data is stored, retrieved, and sorted.
-
Requires in-depth analysis of design alternatives when choosing the most efficient method of storing medical record data.
-
Requires a brand new command to be created and existing code to be edited.
-
-
-
Minor enhancement: added a check to verify if a given date is valid and in the correct format.
-
Code contributed: [Functional Code][RepoSense]
-
Other contributions:
-
Project management:
-
Managed releases
v1.1
-v1.4
(4 releases) on GitHub.
-
-
Enhancements to existing features:
-
Documentation:
-
Community:
-
2. Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
2.1. Displaying medical records of patients : select
Displays all the medical records of the specified person.
Format: select INDEX
Examples:
-
select 1
Displays the medical records of the first person on the displayed list as shown in the figure below.
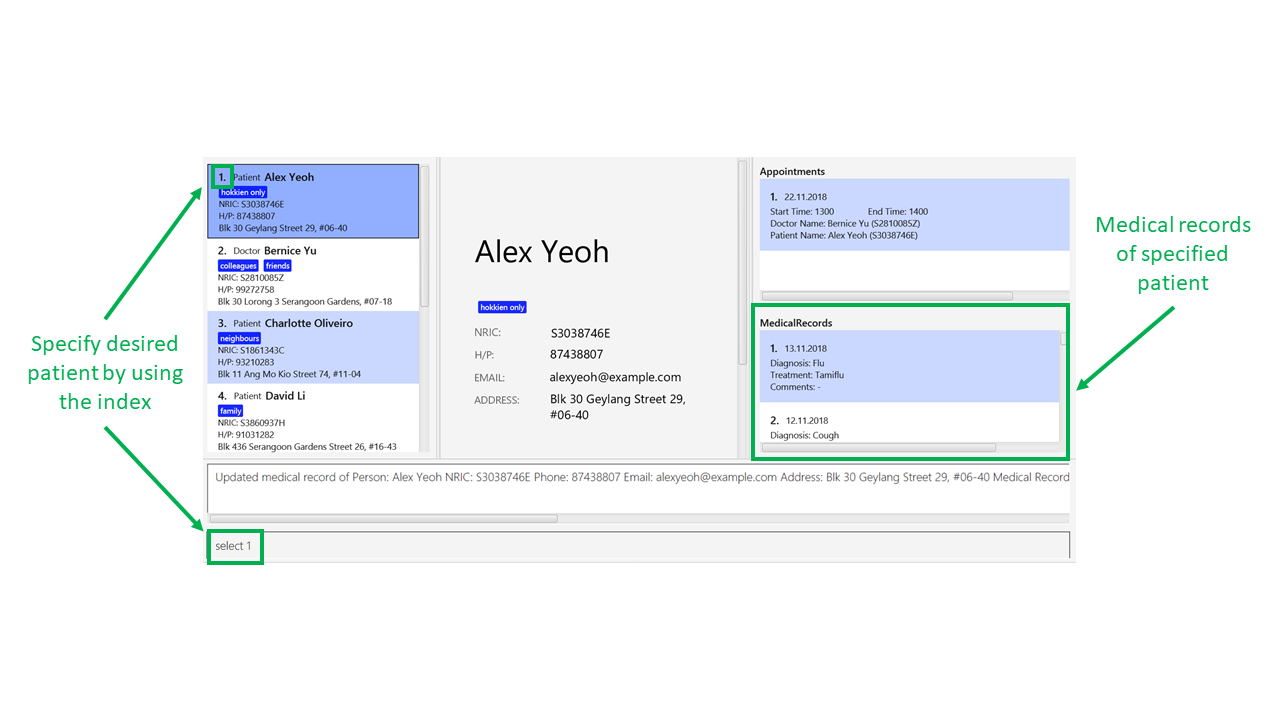
2.2. Updating medical records of patients : update
Adds a new medical record for the patient as the most updated record using the specified date as date of record.
Format: update INDEX d/DATE dg/DIAGNOSIS tr/TREATMENT [c/COMMENTS]
If no comments are specified, "-" will be displayed on the medical record. |
Examples:
-
update 1 d/13.11.2018 dg/Flu tr/Tamiflu
Updates the medical records of the patient at index 1 with the given date, diagnosis and treatment without any comments as shown in the figure below.
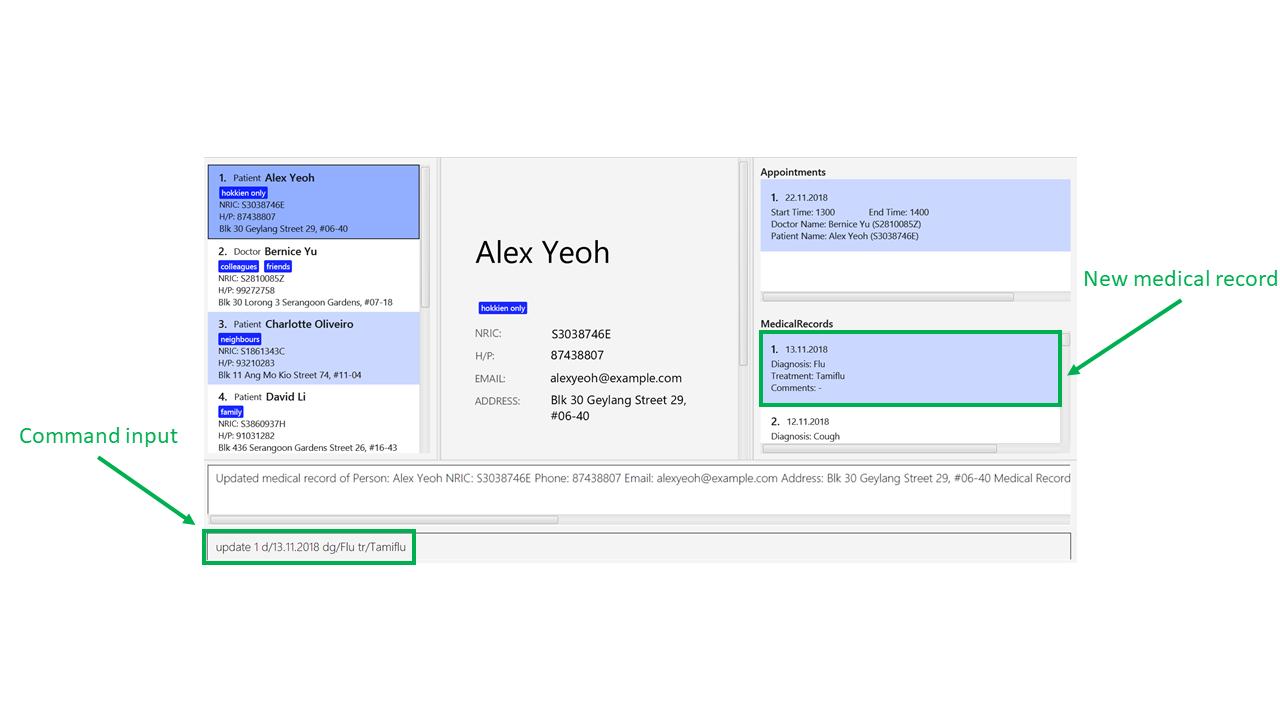
-
update 1 d/13.11.2018 dg/Flu tr/Tamiflu c/To be taken thrice a day after meals.
Updates the medical records of the patient at index 1 with the given date, diagnosis, treatment and comments as shown in the figure below.
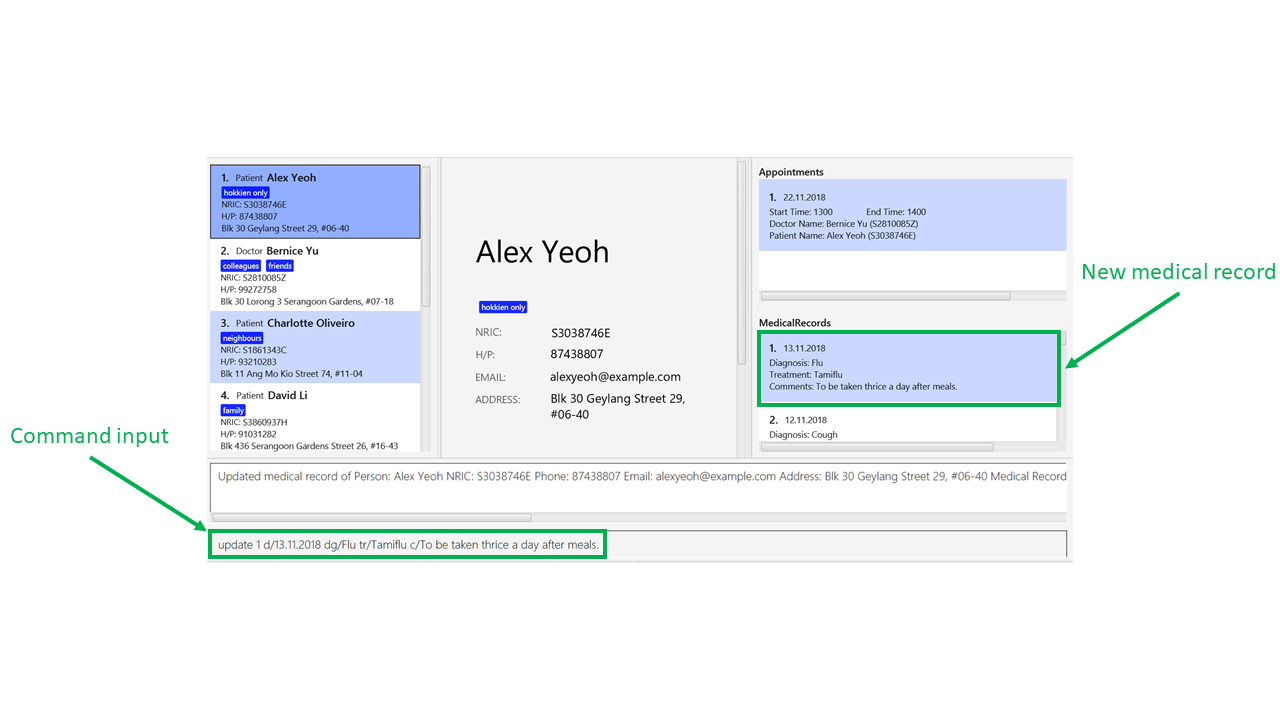
3. Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
3.1. Update command feature
3.1.1. Current implementation
The updating medical record mechanism is facilitated by UpdateCommandParser
, UpdateCommand
and MedicalRecord
.
UpdateCommand
extends from Command
and UpdateCommandParser
extends from Parser
.
The key operation implemented is UpdateCommand#execute
.
Given below is an example usage scenario and how the update command mechanism behaves at each step.
Step 1. The user wants to update the medical record library of a Patient
. User proceeds to fill in the details behind the respective prefixes.
For example: update 1 d/16.10.2018 dg/flu tr/tamiflu c/to be taken thrice a day
Step 2. The user executes the command which calls the UpdateCommandParser
.
UpdateCommandParser
will first parse the inputs and create a MedicalRecord
with the given date, diagnosis, treatment and comment, and return an UpdateCommand
with the new MedicalRecord
.
If no comment is given the default comment "-" is used. |
Step 3. UpdateCommand
will then be executed.
UpdateCommand
will first do the following checks on the user input to ensure that the command input was valid:
Checks:
-
Whether the index of input person is valid.
-
Whether the person specified by the index is a patient.
-
Whether the specified date is a valid date.
-
Whether the specified date is before the current date.
-
Whether the input contain invalid prefixes.
If it was invalid, an exception message will be thrown and the MedicalRecord
will not be added into the patient’s medical record library.
Step 4. If the checks are successful, all the attributes of the specified person will first be copied, including the existing medical record library of the specified Patient
.
Step 5. The new MedicalRecord
will be added into the copied medical record library which is an ArrayList
of MedicalRecord
. The new MedicalRecord
is added to the front of the list and since the pointer for the latest medical record is always pointing to the first element, the latest medical record will be automatically updated.
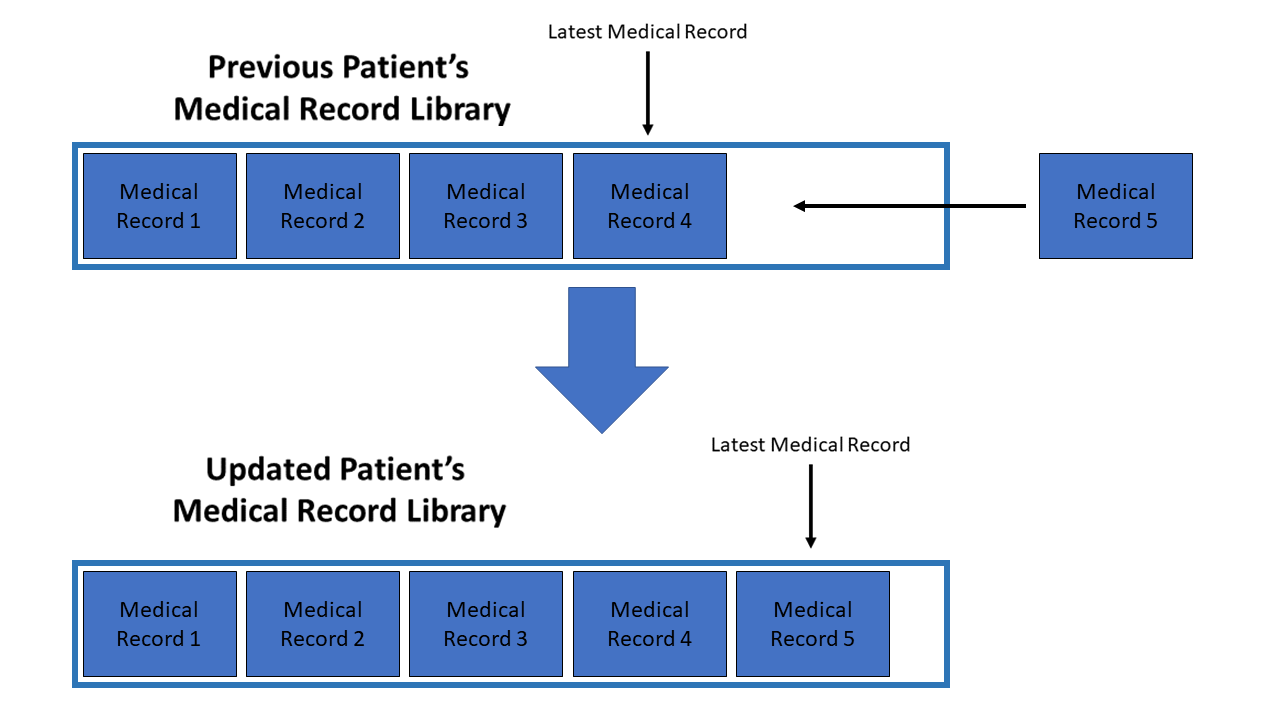
Step 6. A new Patient
is created with all the copied attributes and the updated medical record library.
Step 7. The existing Patient
that was specified is updated to the newly created Patient
in the model.
The following sequence diagram shows how the update medical record operation works:
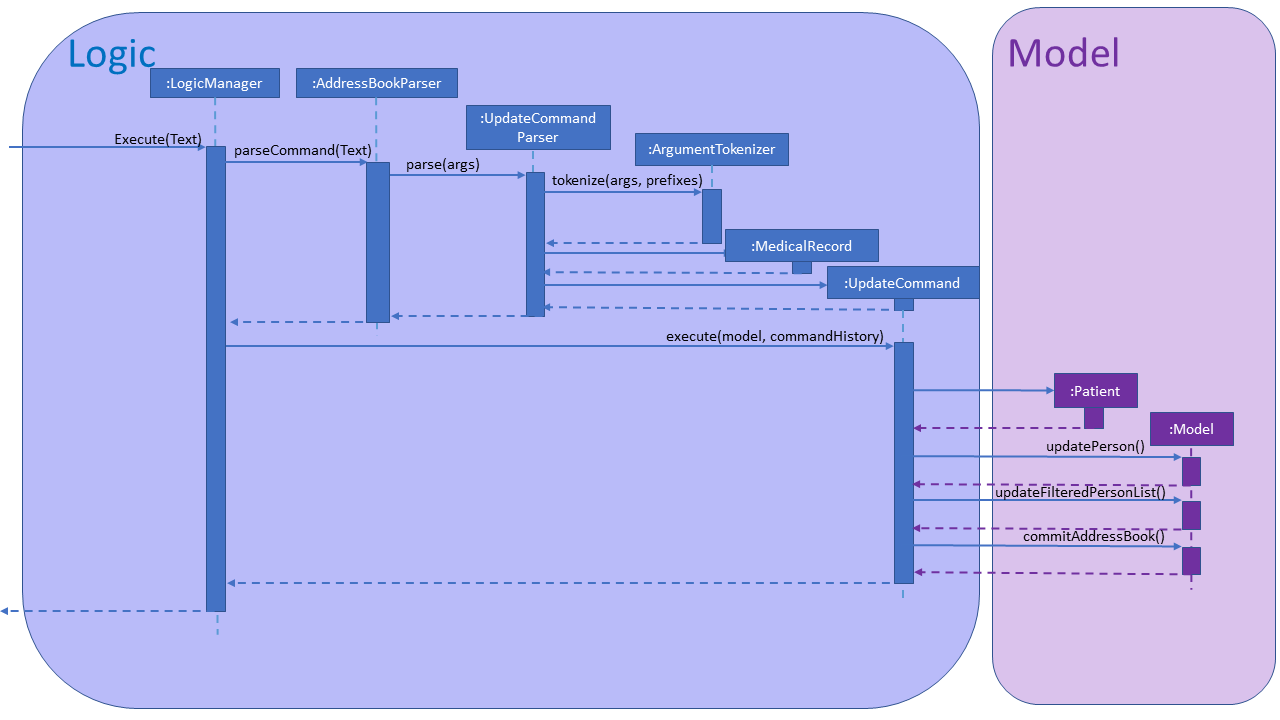
3.1.2. Design considerations
This section describes the pros and cons of the current and other alternate implementations of updating medical records.
Aspect: How to store a patient’s medical record library
-
Alternative 1 (current choice): Use an ArrayList of
MedicalRecord
as the patient’s medical record library.-
Pros: Accessing the most recent record by retrieving the first
MedicalRecord
in the ArrayList is easy. Given below is a code snippet that demonstrates how this is done.if (medicalRecordLibrary.size() != 0) { return medicalRecordLibrary.get(medicalRecordLibrary.size() - 1); } else { return null; }
-
Cons: Retrieving the latest
MedicalRecord
before a specified date takes longer as eachMedicalRecord
has to be checked sequentially until the lastMedicalRecord
before the specified date is found.
-
-
Alternative 2: Use a different data structure like a TreeMap of
MedicalRecord
as the value and date for the key as the patient’s medical record library.-
Pros: Retrieving the latest
MedicalRecord
before a specified date is made convenient by just using the floor function already defined in the TreeMap API. -
Cons: Maintaining the TreeSet for more complicated tasks like editing
MedicalRecord
may be difficult since a newMedicalRecord
has to be created and reinserted into the same location in the TreeSet.
-